40+ Valuable Links To Help You Understand Web3 Stack In 2022
Web3 stands for a new decentralized dimension of the Internet. So far, it’s still in its infancy, and no one has seen it fully deployed and functioning yet. However, we already have many prospective projects and solutions that call themselves Web3 related, and we also have some basic understanding of the Web3 stack.
Some time ago, we discussed how to become a Web3 developer.
In this guide, I will walk through some Web3 stack elements in more detail and focus on the programming languages and tools used in the current Web3 development.
Grab your coffee and enjoy the reading. ☕️
Start of Web3 programming: Bitcoin and C++
The first public blockchain Bitcoin was written in C++
The choice of this language was not random: C++ served perfectly for Bitcoin’s multithreading model.
Essentially, multithreading refers to the capability of a system to divide its tasks into multiple individual threads and run them simultaneously in parallel. In the end, it helps achieve multitasking — higher performance and productivity for a short time.
Examples of multithreaded parallel operations in Bitcoin are verification of addresses, checking digital signatures, etc.
In contrast, a single threading system implies the execution of only a single task at a time without interruption, which is time-consuming and less productive.
Other merits of C++ as a blockchain programming language were
- its maturity in terms of upgrades and debugging, and
- the dynamic memory management model via move semantics and forwarding, which allows developers to fetch objects without creating copies of temporary objects — this improves the runtime execution speed.
Although C++ matched well with the functionality of the Bitcoin network, it was not sufficient for other types of blockchains, whose stacks included the elements that were not present or well-developed in Bitcoin — for example, smart contracts.
Generally, the proper programming language for the blockchain stack is the one that at least provides for:
- a compact code size — as we know, blockchain storage is expensive, and code size has to be space-saving.
- an increased level of security
- proper auditing and debugging capabilities, and
- deterministic way of doing things, which implies that every event or action that takes place is purely due to a set of previously happened events or actions.
Given this, let’s look at particular programming languages primarily used in Web3 development.
Smart contracts programming languages
With the popularity of Bitcoin, the concept of “programmable money” and peer-to-peer payments very soon got onto the next level of development. It was proposed that blockchain transactions run through smart contracts — self-executing pieces of code that provide complete transparency and eliminate human interference.
For accuracy, the idea of smart contracts was introduced more than a decade earlier than the rise of crypto. In 1994 Nick Szabo, an American computer scientist and cryptographer, proposed “smart contracts” as computerized transaction protocols wired to guarantee tamper-proof execution of agreements. According to Szabo, the general objectives of smart contract design are to satisfy common contractual conditions (such as payment terms, liens, confidentiality, and even enforcement), minimize exceptions, both malicious and accidental, and minimize the need for trusted intermediaries.
However, the way we know them now, smart contracts gained their popularity later on with the introduction of Ethereum. For the purpose of smart contracts coding, Ethereum’s team developed a completely new programming language — Solidity.
Solidity
It is a high-level object-oriented programming language created by the Ethereum Network team for building and deploying smart contracts. Solidity was designed to target the Ethereum Virtual Machine (EVM) — a software platform that constitutes the core of the Ethereum blockchain and enables developers to create various types of programs and applications (dApps) using smart contracts.
EVM has all features of a Turing-complete virtual machine, which means it can execute any type of code exactly as intended. In other words, Ethereum’s Turing Completeness implies that it can use its codebase to perform virtually any task, as long as it has the correct instructions, enough time, and processing power.
Solidity is syntactically similar to JavaScript, C++, and Python, which makes it easy for coders to master.
Solidity is statically typed and supports inheritance, libraries, and complex user-defined types, among other features.
With Solidity, one can create contracts for voting, crowdfunding, blind auctions, multi-signature wallets, etc. The scope of its application is unprecedented.
Solidity is used almost in all EVM-compatible networks (Polygon, BSC, Avalanche, RSK, Fantom, Telos, etc.) and in most Layer 2 scaling solutions, like Arbitrum, Optimism, Zk sync, Parastat, and others.
Solidity is the most widely used language for smart contracts and has the largest dev community compared with other smart contract languages.
Rust
Rust is a low-level programming language used for smart contract coding in such prominent blockchains as Solana, Polkadot, Near, and others.
Rust is memory efficient and statically typed. Unlike Solidity, Rust is a general-purpose language, and smart contracts are just one of the use cases of its application. One of Rust’s biggest advantages as a blockchain programming language is that it allows the creation of a code that doesn’t have memory bugs and consumes less storage on the blockchain.
Among other valuable features that Rust offers are type safety, small runtime, no undefined behaviors, and the like. Rust’s compiler is ergonomic and provides color-coded outputs and detailed error reports.
Rust is the second most used language for smart contracts.
Vyper
According to its documentation, Vyper is a contract-oriented, pythonic programming language that targets the Ethereum Virtual Machine (EVM). The main goal behind Vyper is to simplify smart contracts, provide their superior auditability and make them less vulnerable to attacks. So Vyper is contemplated as an upgraded, more user-friendly, and secure version of Solidity. Vyper aims to make it virtually impossible for developers to write misleading code.
Yul
Yul (previously also called JULIA or IULIA) is a simple, low-level intermediate language for the Ethereum Virtual Machine. The Solidity Developers wrote Yul as a compilation target for further optimizations. It features simplistic and functional low-level grammar. It allows developers to get much closer to raw EVM than Solidity, and with that comes the promise of drastically improved gas usage.
Yul was recently upgraded to Yul+ extension.
Yul is a language that hasn’t seen much popularity yet, however it has tons of potential for future use.
Plutus
Plutus (Haskell) is a statically typed programming language wired for writing reliable smart contracts on the Cardano blockchain. It is also a functional programming language, which means programs are composed as sets of mathematical functions for execution.
Python
Python is a general-purpose programming language with a readable code design philosophy. It is especially gaining popularity for data analysis and neural network programming.
Python is also used for smart contract programming. A notable example here is Algorand, a public blockchain in which smart contracts are written in Python using PyTeal library.
Others
Languages like Go Lang, Rholang, and Javascript are also often used as blockchain programming languages. However, unlike Solidity and Rust, they do not apply as languages for writing smart contracts. Instead, these languages can be used for creating environments to interact with blockchains (like a JavaScript environment) through smart contract call functions.
So here is a summary of the smart contract programming languages covered above and the blockchain networks where they apply:
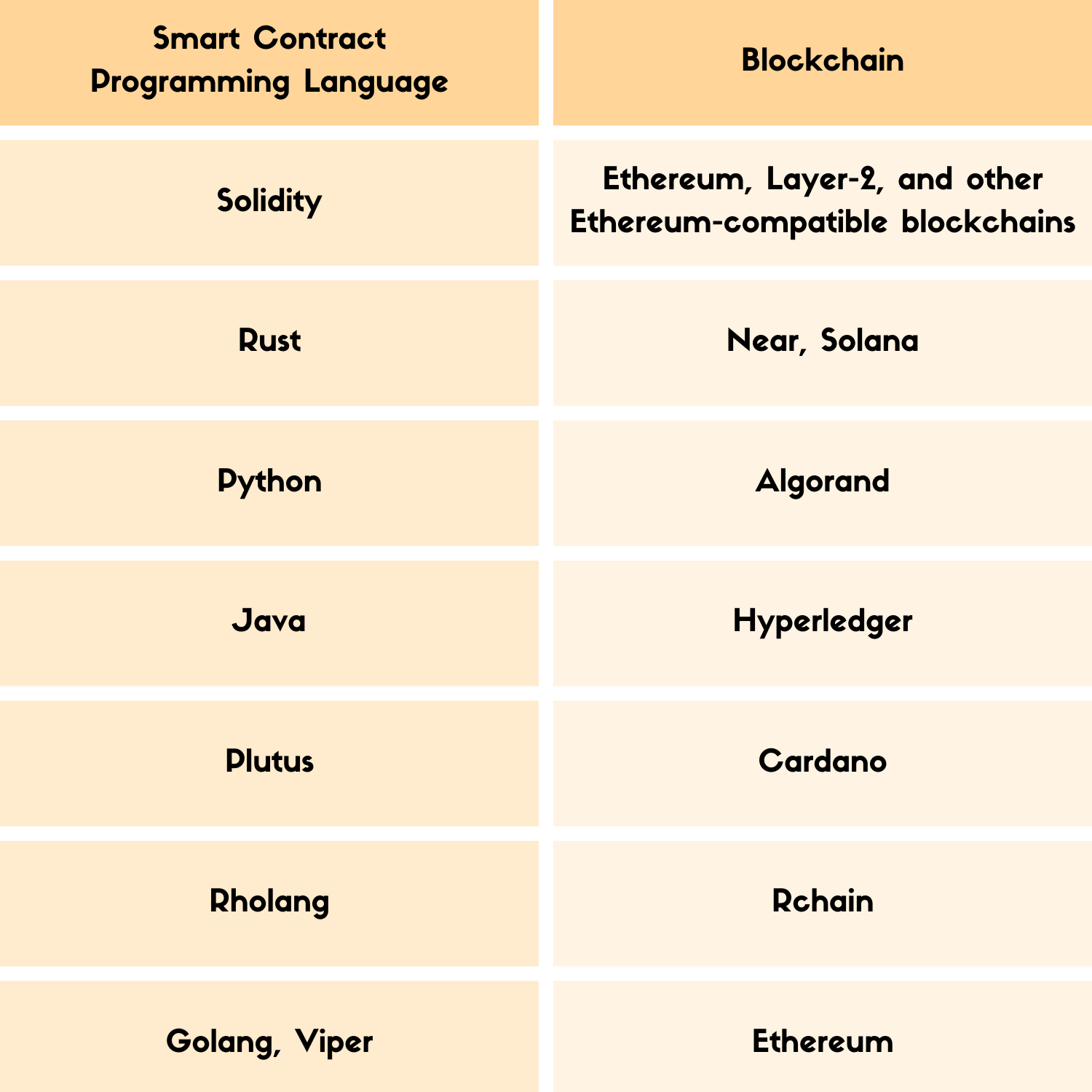
Web3 Tools
First, let’s look at the main building blocks of the Web3 stack, which are represented in the table below.
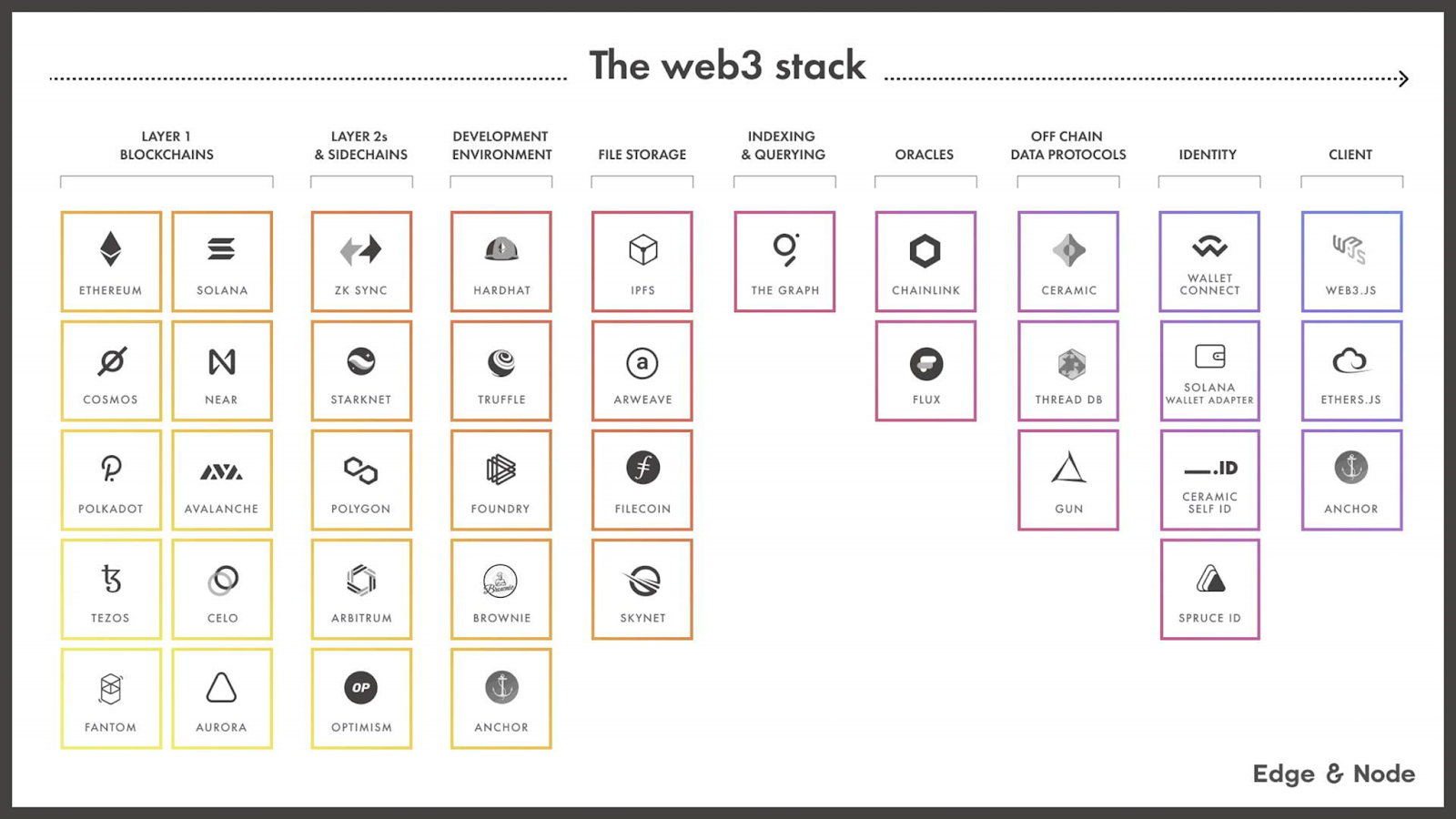
Now, let’s look at some of these blocks closer.
Web3 IDEs/SDKs
Both SDKs (Software Development Kits) and IDEs (Integrated Development Environment) are collections of various tools in one installable package used by developers to create applications for specific platforms or purposes. They are designed to simplify and automate the process of development. With these tools, there is no need to code everything from scratch.
The main difference between SDKs and IDEs is that IDEs are purposed to provide an interface for writing and debugging codes, while SDKs are used to add functionality to the codes written.
The actual contents of IDEs and SDKs vary from one to the other. They can include libraries, frameworks, documentation, preprogrammed code pieces, API, testing/debugging tools, etc. Some SDKs have a dedicated IDE that one can use right out of the box.
Here are some popular Web3 IDE/SDK examples:
IDEs
- Hardhat and Truffle — terminal-based IDEs that allow developing, testing, and deploying smart contracts on the Ethereum blockchain. Though they have some slight differences, the functionality of these two IDEs is pretty similar. Both provide JavaScript and Solidity-based development frameworks.
- Remix — a browser-based IDE serving similar purposes as Hardhat and Truffle. Remix IDE allows developers to write smart contracts in Solidity from web browsers and desktop apps. It has modules for testing, debugging, and deployment, and a diverse set of plugins with intuitive GUIs.
- Ganache — allows you to set up your personal blockchain to test smart contracts and dapps. It’s a kind of a simulator of Ethereum blockchain that makes developing Ethereum applications faster, easier, and safer.
SDKs
SDKs contain libraries, documentation, and sample codes to make developers’ lives easy. For instance, directly plugging into an RPC (Remote Procedure Call) node (i.e., a remote node facilitating communication between blockchain and dApps) can be complicated and time-consuming. This is where SDKs might come in handy.
web3.js, web3.py, and ethers.js are the most popular Web3 SDKs (libraries’ collections). They allow connecting smart contracts with the front end of an application. In other words, these tools would help turn your web application into a Web3 one.
Niche Web3 SDKs
By that sort of SDKs, I mean those specifically wired for building certain types of applications.
Here is an example. Let’s say you want to launch a crypto exchange or a brokerage platform. For that, you can use the OpenDAX WEB SDK — a part of comprehensive OpenDAX software wired for establishing crypto exchanges and brokerage apps.
OpedDax WEB SDK provides the developers with all necessary react components and tools, allowing them to launch a Web3 exchange within days without spending hundreds of thousands of dollars and months of work on the software and web development.
Blockchain APIs
Blockchain APIs (application programming interfaces) allow dApps to communicate with blockchain networks.
APIs are used in many crypto-related areas: payments, trading, clearing, analytics, data management, account management, and the like.
Many blockchain APIs are available open-source and come as IDE/SDK package components. For example, the web3.js mentioned above enables interaction with a local or remote Ethereum node using HTTP, IPC, or WebSocket.
Another example is ethers.js — a JavaScript API for interacting with the Ethereum blockchain. While web3.js assumes that there is a local node connected to the application that stores keys, signs transactions, and interacts with the blockchain, Ethers.js separates these functions between a wallet that holds the key and a provider that serves as a connection to the network, checks the state and sends transactions. This approach gives more flexibility to developers.
Here are a couple of more blockchain API examples:
- Graph — a decentralized querying and indexing protocol from Ethereum and IPFS. It allows developers to build on open APIs called sub-graphs.
- Alchemy Web3 APIs. According to its documentation, Alchemy Web3 is a wrapper around Web3.js, providing enhanced API methods and other crucial benefits listed below. It is designed to require minimal configuration so you can start using it in your app right away.
Distributed data storage solutions
As we know, one of the key things that Web3 aims to procure is the decentralized, secure, and immutable storage of data.
What does this mean?
The vision is to ensure that users’ information is stored sliced to multiple independent network nodes instead of one server that is not under users’ control. This distributed approach will procure high-quality data protection, as well as enable users to manage their data, encrypt and keep it private, authorize access to data, and backup the storage.
The most notable projects that work in this direction are:
Block explorers
A block explorer is an online blockchain browser that tracks transactions on a particular blockchain. It publicly shows such transaction details as TXID, amount, timestamp, etc.
Also, block explorers provide APIs to access their data by dApps.
Examples:
Oracles
A blockchain oracle is software that facilitates communication between blockchains and any off-chain system, including data providers, web APIs, enterprise backends, cloud providers, IoT devices, e-signatures, payment systems, and other blockchains, etc.
Oracles work both on and off the blockchain simultaneously. On-chain oracles establish a blockchain connection (handle requests), broadcast data, send proofs, extract blockchain data, and perform computation. Off-chain oracles process requests, retrieve and format external data, send blockchain data to external systems, and perform off-chain computation for greater scalability, privacy, security, and other smart contract enhancements.
Examples here:
Automation tools
Here, we mean the tools to create and automate Web3 applications. An example here is Open Zeppelin which has a library of reusable audited smart contracts for multiple use cases such as DeFi, NFTs, and DAOs. It also has automation tools for smart contract operations and scripts to call smart contract functions.
Smart contract audit tools
One of the biggest challenges with Web3 applications and driving them smart contracts is that they are such irresistible magnets for hackers and attackers. This is why auditing is a non-negotiable part of any dApp/smart contract development and deployment. Auditing helps identify potential vulnerabilities of smart contracts, their backdoors, bugs (if any), and other security flaws that must be eliminated at all costs before a dApp starts widely applying. Auditing also allows finding out some gas optimization opportunities for a contract, making it more cost-effective.
The audit is usually done by third-party companies specializing in it. Here are some examples of tools these guys apply in their activity:
- Certik — offers tools for security audit, transaction monitoring and insights, wallet tracing and visualization, and attack simulation.
- Consensys Diligence — provides automated testing and verification tools.
- Sliter is a static smart contract security analytic tool built on Python to detect vulnerabilities, enhance code comprehension, and prototype custom analyses.
- MythX, Mythril, Manticore, and Echidna are other tools for security audits.
Data analytics tools
Anyone can access public chain data and run analytics. Web3 is currently in the early stages, wherein decentralized applications are still being developed. As these applications scale and mature, analytics will play a crucial role in keeping the ecosystem up, running, and safe.
Dune analytics provides tools to query, extract and visualize data from public blockchains. Querying is done with SQL. Dune currently supports Ethereum, Binance Smart Chain, Polygon, Gnosis chain, and Optimism.
TRM Labs and Chainalysis have a suite of paid analytics products for forensics and transaction analytics.
No-code tools
Today no-code development in the blockchain is gradually gaining traction. No-code is poised to enable the creation dApps and other solutions easily and effortlessly, without having special knowledge or hard skills.
With no-code tools, one can create and launch tokens, Decentralized Autonomous Organizations (DAOs), NFT marketplaces, NFT minting platforms, Generative Art, etc.
Examples:
- Third Web — a platform for launching NFT marketplaces and tokens,
- Mirror.xyz: — a platform for tokens minting, governance, and NFT marketplaces. Mirror positions itself as the essential web3 toolkit for sharing and funding anything, and
- Aragon — an open-source infrastructure with governance plugins for creating DAOs.
Multisig wallets
A multisig wallet is a digital wallet that requires more than one private key to sign and authorize a crypto transaction. In some cases, it assumes that several different keys must be used to generate a single required signature.
A multisig wallet can be of the m -of- n type where any m private keys out of a possible n are required to sign and approve a transaction. The main purpose of multisig wallets is to protect funds from being misused or stolen.
Multisig wallets are popular among all types of crypto market players: retail investors, crypto exchanges, investment funds, brokers, OTCs, DAOs, etc.
Advantages of multisig wallets:
- Increased security: a hacker needs to crack at least m-keys instead of just one to access an account and move funds from it.
- Assets recovery: if some of the n-keys are lost, the funds can still be accessed through the remaining keys.
- Collective control over funds: Funds can not be withdrawn without the unanimous consent of all authorized team members who are in charge of funds management.
Multisig wallets examples:
- Gnosis Safe
- BitGo, and
- Armory.
Web3 starter kits
For example, Scaffold ETH helps quickly start building and prototyping on the Ethereum blockchain. It provides tutorials and libraries to make DeXs, NFTs, multisig wallets, smart contracts, etc.
Support forums
Forums could be a great additional help on the Web3 development journey if you get stuck and need help. The most popular ones are Stack overflow, Ethereum stack exchange, as well as different thematic Discord and Reddit threads.
Final word
Web3 stack has been developing sporadically and is quite fragmented so far. Apparently, it’s still in its baby stage, and so is its knowledge base. However, the speed at which the Web3 development moves is pretty comparable to lighting.
Already today Web3 is no longer the exclusive territory of a bunch of dedicated techies. It provides an impressive toolkit that does not require us to be PhDs in computer science but instead leaves room for quick learning and active participation in the new Internet ecosystem development. It also allows us to harness the accumulated knowledge of Web2, so we can avoid reinventing the wheel each time trying to make things differently.
Sooner or later, even amateurs would be able to find their place under the Web3 sun. The entry barriers are becoming lower, so the more people passionate about this space will be able to join without being dismantled by its complexity.
Want to learn more about Web3 and FinTech?
Follow us for more Web3 stories from Yellow powered by Openware!
- Check out the most ambitious decentralized project, Yellow Network P2P financial info exchange powered by the latest state channel technology.
- Take a look at the open-source OpenDAX v4 white-label cryptocurrency exchange software stack on GitHub.
- Follow Yellow on Twitter.
- Join the Yellow Network Telegram.
- Read more exciting articles on Yellow Network Medium blog.
- Find Yellow Network on Hacker Noon.